Using Terraform with Azure Key Vault to Retrieve Secret Values
In this blog post we will explore how to use Terraform, with Azure Key Vault to retrieve secret values.
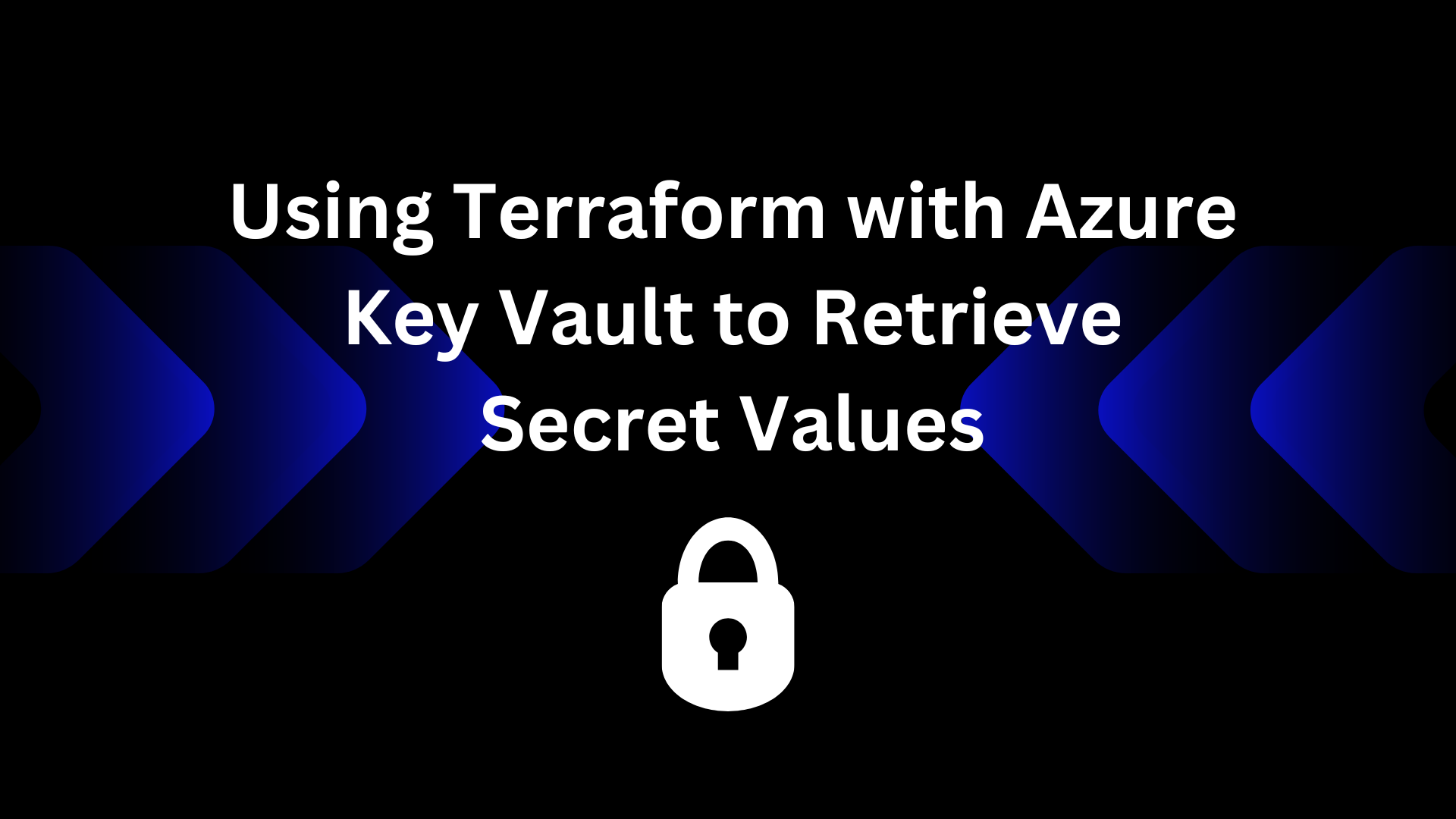
In this blog post, we will explore how to use Terraform, with Azure Key Vault to retrieve secret values.
What is Azure Key Vault?
Azure Key Vault is a cloud service provided by Microsoft, designed to safeguard and manage cryptographic keys, secrets, certificates, and other sensitive information used by cloud applications and services.
Operating as a scalable and fully managed solution, Azure Key Vault alleviates the complexities of key management, enabling organisations to easily generate, store, and control access to encryption keys and secrets, thus enhancing the security posture of their digital assets.
Prerequisites
Before we being, make sure you have the following:
- An Azure account
- Terraform installed and configured on your local machine
Step 1: Setting up Azure Key Vault
We are going to deploy an Azure Key Vault using Azure CLI. We will use the Azure Cloud Shell to do this.
- Head over to https://shell.azure.com
- Ensure you are in a Bash session
- Create a new resource group by using the command:
az group create --name techielassrg --location eastus
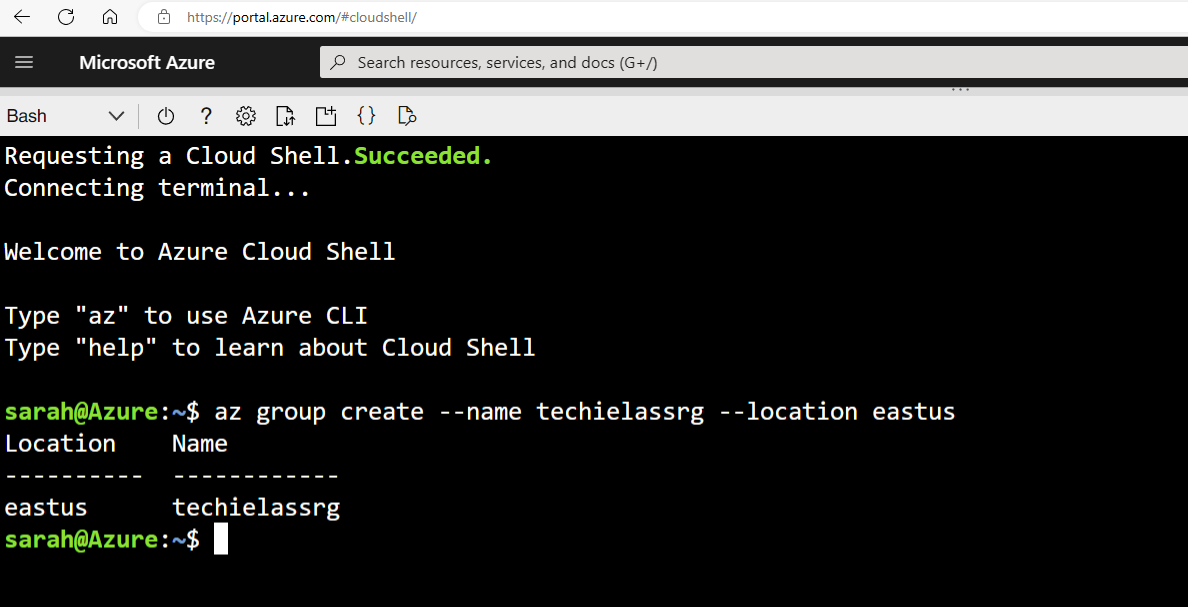
- With the resource group created we can now create the Key Vault:
az keyvault create --name "Techielasskeyvault" --resource-group "techielassrg" --location "East US"
A basic Key Vault is now created.
Step 2: Create a secret in the Azure Key Vault
With the Key Vault now created the next step is to create a secret within it we can retrieve from our Terraform configuration.
Still with the the Azure Cloud Shell type in the command:
az keyvault secret set --vault-name "techielasskeyvault" --name "techielass-secret" --value "scotlandrules"
Step 3: Retrieve an Azure Key Vault secret using Terraform
We now have an Azure Key Vault configured and a secret stored within it. It’s time to start writing the Terraform file we will use to retrieve the secret.
Opening up my favourite editor, VS Code I create a new file and type in the following:
provider "azurerm" {
features {}
}
data "azurerm_key_vault" "existing" {
name = "techielasskeyvault"
resource_group_name = "techielassrg"
}
The above section tells Terraform we are interacting with Azure. Then it says we are using a data source outside of Terraform, which in this case is our existing Azure Key Vault.
The next section we add to our Terraform file is:
data "azurerm_key_vault_secret" "example" {
name = "techielasssecret"
key_vault_id = data.azurerm_key_vault.existing.id
}
This is instructing Terraform to retrieve a specific secret from our key vault.
The last section for the Terraform template is:
output "secret_value" {
value = nonsensitive(data.azurerm_key_vault_secret.example.value)
}
This Terraform configuration tells Terraform to output the secret once it has retrieved it to our command line console.
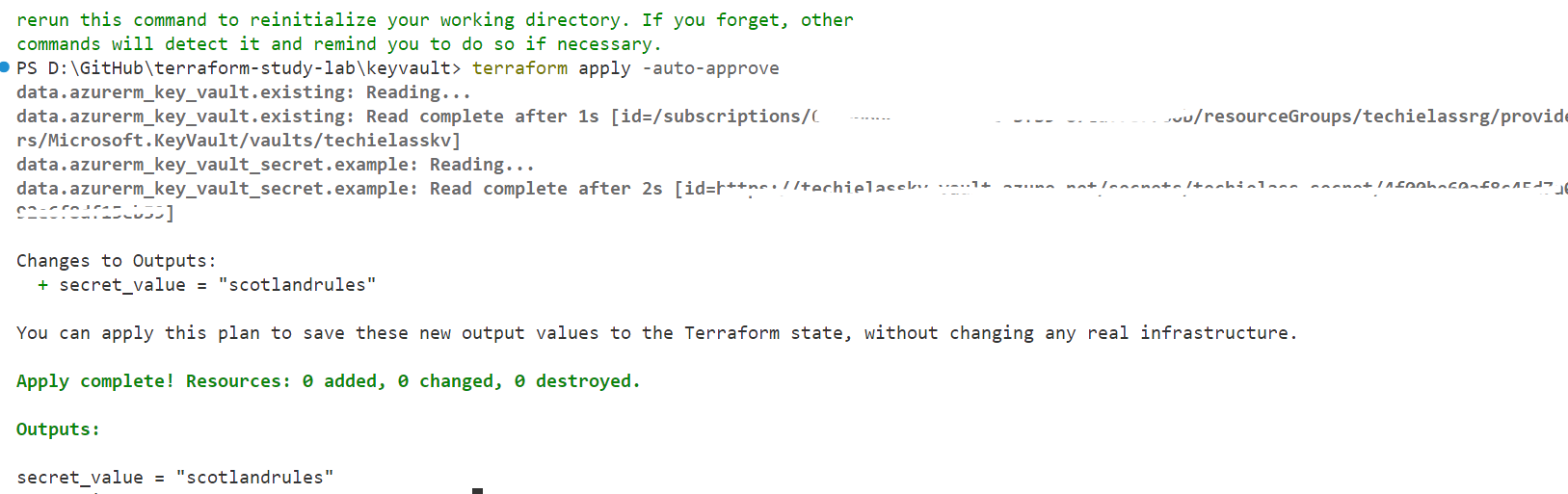
You’ll notice on the value line I have encased the value I want to display within nonsensitive() this is so we can see the secret output within the command line.
If we didn’t do this then we would get an error as Terraform tries to avoid sensitive data being displayed.
Step 5: Initialize and Apply Terraform Configuration
To complete the process we need to issue to commands:
terraform init
terraform apply –auto-approve
This will download the necessary provider plugins then apply the configuration.
Once the commands run you will see the secret displayed.
Step 4: Deploy an Azure resource using Terraform and retrieve an Azure Key Vault secret
Now that we have gone through the process of creating a key vault, storing secrets and retrieving it via Terraform it’s time to use this new knowledge into a practical example.
We will create an Azure storage account using Terraform and the name of the storage account will be the secret value we created.
The following Terraform template will retrieve the secret, create a new resource group and then create an Azure storage account.
provider "azurerm" {
features {}
}
data "azurerm_key_vault" "techielasskv" {
name = "techielasssecrets"
resource_group_name = "techielassrg"
}
data "azurerm_key_vault_secret" "techielasssecret" {
name = "techielass-secret"
key_vault_id = data.azurerm_key_vault.techielasskv.id
}
resource "azurerm_resource_group" "rg" {
name = "techielassstorage"
location = "eastus"
}
resource "azurerm_storage_account" "techielasssa" {
name = data.azurerm_key_vault_secret.techielasssecret.value
resource_group_name = azurerm_resource_group.rg.name
location = azurerm_resource_group.rg.location
account_tier = "Standard"
account_replication_type = "LRS"
depends_on = [
azurerm_resource_group.rg
]
}
Once you deploy this Terraform file, you should have a storage account with the name of the secret value we created earlier.
Conclusion
In this blog post, we’ve explored how to use Terraform with Azure Key Vault to retrieve secret values.
This approach provides a secure and efficient way to manage secrets within your cloud environment and deployments.
Remember to always keep your secrets secure and never expose them within your code or version control systems.